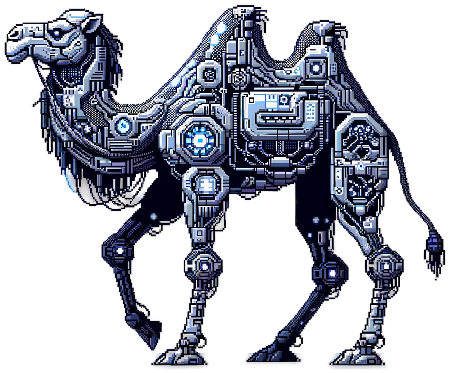
Recursive File Search Magic with IO::All
Perl’s IO::All module is a Swiss Army knife for file operations. Today, we’re exploring one of its most powerful features: recursive file searching. We’ll look at two approaches to find all ‘index.html’ files in a directory tree.
Example 1: The Verbose Approach
Let’s start with a more explicit method:
use IO::All;
my $dir_posts = '/var/www/output/';
# Use the All method (capital A) for recursive search
my @files = io($dir_posts)->All_Files;
my @indexes;
foreach my $file (@files) {
if ($file->filename eq 'index.html') {
push @indexes, $file->absolute->name;
} }
Here’s what’s happening:
- We create an IO::All object for our directory and call All_Files. The capital ‘A’ is crucial - it tells IO::All to search recursively.
- We iterate through each file, checking if its filename is ‘index.html’.
- Matching files have their absolute paths added to @indexes.
This method is straightforward and easy to understand, especially for those new to Perl or IO::All.
Example 2: The Concise Approach
Now, let’s see how we can achieve the same result more succinctly:
my @indexes = grep { $_->filename eq 'index.html' } io($dir_posts)->All_Files;
This one-liner packs a punch:
- io($dir_posts)->All_Files still does the recursive search.
- grep filters the list, keeping only ‘index.html’ files.
- **$_** represents each file as we iterate.
- The result is directly assigned to @indexes.
This approach showcases Perl’s expressive power, combining built-in functions with IO::All methods for concise, readable code.
Both methods have their place. The verbose approach might be preferred for its clarity, especially in larger scripts or when working with less experienced developers. The concise version demonstrates Perl’s capacity for elegant, efficient solutions and is often favored by seasoned Perl programmers.
IO::All’s All_Files method simplifies what would typically require complex recursive functions or use of modules like File::Find. Whether you’re searching for specific files, processing many files, or inventorying a directory, IO::All’s recursive capabilities can significantly streamline your code.
While we’ve focused on All_Files here, IO::All offers many other powerful file and directory operations. It’s definitely worth exploring further to see how it can enhance your Perl file-handling toolkit.
Copyright ©️ 2024 perl.gg