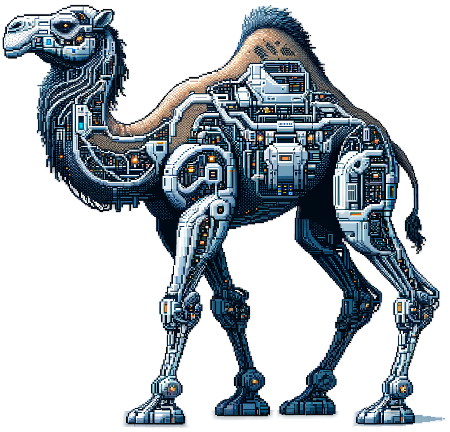
Subroutine Interpolation Magic
Perl’s expressiveness shines in its string interpolation capabilities. Today, we’re exploring how both ${()} and @{[]} can interpolate subroutines - both pre-defined and anonymous. Let’s dive into some examples to see these powerful constructs in action.
Key Differences and When to Use Each
- Use ${()} when you want to force scalar context. Is best for interpolating single values.
- Use @{[]} when you want to preserve list context or when the return value’s context doesn’t matter.
Note: when used in print or say statements, the distinction between scalar and list context becomes less significant.
The ${()} Construct
The ${()} construct is particularly useful when you want to interpolate the result of a subroutine that returns a scalar value. It forces scalar context on the expression inside.
Example with an Existing Subroutine
use feature qw(say);
sub get_greeting
{my $hour = (localtime)[2];
return $hour < 12 ? "Good morning" : "Good afternoon";
}
say "Hello! ${\(get_greeting())}";
This will output either “Hello! Good morning” or “Hello! Good afternoon” depending on the time of day.
Example with an Anonymous Subroutine
use feature qw(say);
my $current_temp = 22; # Celsius
say "The temperature is ${\( sub {
my $fahrenheit = ($current_temp * 9/5) + 32;
return sprintf("%.1f°C (%.1f°F)", $current_temp, $fahrenheit);
}->() )}";
This will output something like: “The temperature is 22.0°C (71.6°F)”
The @{[]} Construct
The @{[]} construct is more flexible and can handle expressions that return lists or scalar values. It’s particularly useful when you want to preserve list context.
Example with an Existing Subroutine
use feature qw(say);
sub get_top_cities {
return ("Tokyo", "Delhi", "Shanghai", "São Paulo", "Mexico City");
}
say "The top 3 most populous cities are: @{[join(', ', (get_top_cities())[0..2])]}";
This will output: “The top 3 most populous cities are: Tokyo, Delhi, Shanghai”
Example with an Anonymous Subroutine
use feature qw(say);
my @numbers = (1, 2, 3, 4, 5);
say "Doubled numbers: @{[ sub {
return map { $_ * 2 } @numbers;
}->() ]}";
This will output: “Doubled numbers: 2 4 6 8 10”
Wrapping Up
Both ${()} and @{[]} offer powerful ways to interpolate the results of subroutines - whether pre-defined or anonymous - directly into your Perl strings. These constructs allow for dynamic, context-aware string generation that can significantly enhance the expressiveness of your code.
By mastering these interpolation techniques, you’ll have yet another set of tools in your Perl toolbox for creating dynamic, expressive strings. Happy Perl programming!
Copyright ©️ 2024 perl.gg