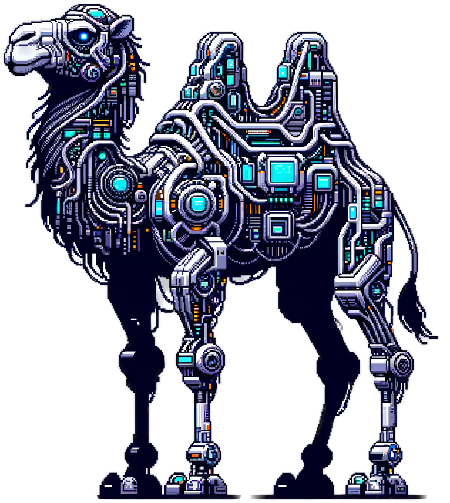
‘do’ Operator: A Comprehensive Guide
Perl’s ‘do’ operator is a versatile tool that often confuses newcomers and even some experienced programmers. In this post, we’ll explore its various uses, from loading code to creating conditional and looping structures. By the end, you’ll have a comprehensive understanding of this powerful Perl feature.
Loading Multiple Subroutines from Files
Let’s start with a common scenario: loading multiple subroutines from a directory of Perl files:
my $dir_subs = './lib/';
foreach my $file (glob("${dir_subs}*.pl")) {
my $result = do($file) or die "Cannot load $file: $@\n";
}
This code:
- Iterates through all .pl files in the $dir_subs directory.
- Uses ‘do’ to load and execute each file.
- Any subroutines defined in these files become available in the current scope.
- The $result variable is mainly used for error checking.
For this to work, your .pl files should define subroutines normally:
# In ./lib/math_functions.pl
sub add {
my ($a, $b) = @_;
return $a + $b;
}
sub multiply {
my ($a, $b) = @_;
return $a * $b;
}
1; # Don't forget this!
Note: That 1; at the end of Perl modules isn’t just tradition. It serves a practical purpose: returning a true value when the module is loaded. When you do a file, this 1 gets assigned to the receiving variable, confirming successful execution. While modern Perl is more forgiving, keeping this convention helps with debugging and maintains consistency. It’s a simple yet effective way to ensure your module loaded without errors and keeps your code in line with community standards.
After running the loading code, you can use these subroutines directly:
print add(5, 3); # Outputs: 8
print multiply(4, 7); # Outputs: 28
Loading a Single Anonymous Function
Now, let’s dive into a more esoteric use of ‘do’ for loading individual anonymous functions:
my $func_name = 'square';
my $func = do "./subs/$func_name.pl";
print $func->(4) if ref $func eq 'CODE'; # Outputs: 16
This method is distinctly different from loading multiple named subroutines:
- It loads a file containing just one anonymous subroutine.
- There’s no need for a ‘1;’ at the end of the file.
- The entire content of the file is the anonymous sub definition.
Here’s what the content of “./subs/square.pl” looks like:
# Content of ./subs/square.pl
sub {
my ($n) = @_;
return $n * $n;
}
Key points to understand:
- The file contains only an anonymous subroutine definition.
- When you ‘do’ this file, it evaluates the anonymous sub definition.
- The last (and only) expression in the file is this anonymous sub, which becomes the return value of ‘do’.
- This returned code reference is assigned to $func.
This pattern allows you to:
- Store individual functions in separate files.
- Load only the functions you need.
- Avoid polluting the global namespace with function names.
Creating Ad-hoc Code Blocks with do
The ‘do {}’ block is another use of the ‘do’ operator:
my $result = do {
my $x = 5;
my $y = 7;
$x * $y; # This is the return value
};print $result; # Outputs: 35
The do {} block:
- Creates a self-contained scope.
- Executes the code inside immediately.
- Returns the value of the last expression in the block.
It’s useful for complex initializations or creating multi-statement expressions.
Conditional Execution with do if
The ‘do’ operator can be used with ‘if’ for conditional execution:
do {
print "It's sunny!";
open_windows();
turn_off_heater();if $weather eq 'sunny'; }
This structure:
- Executes the block only if the condition is true.
- Is useful for grouping multiple statements under a single condition.
- Can be more readable than a multi-line if block for simple cases.
Looping with do while
‘do’ can create a do-while loop, which always executes at least once:
my $i = 0;
do {
print "i is $i\n";
$i++;
while $i < 5; }
Key points:
- The loop body executes before the condition is checked.
- Guarantees at least one execution of the loop body.
- Useful when you need to perform an action before testing a condition.
Loading and Executing External Files
‘do’ can load and execute external Perl files:
my $result = do "/path/to/file.pl";
die "Couldn't parse file: $@" if $@;
die "Couldn't do file: $!" unless defined $result;
This usage:
- Loads and executes the specified file.
- Returns the value of the last expression executed in the file.
- Is useful for dynamically loading configuration or plugin files.
Creating Inline Subroutines
You can use ‘do’ to create and immediately execute an anonymous subroutine:
my $result = do {
my $x = 10;
sub { $x * 2 }
}->();print $result; # Outputs: 20
This technique:
- Creates a closure with its own scope.
- Immediately invokes the anonymous sub.
- Is useful for complex one-off computations.
Key Differences Summarized:
- Loading multiple subroutines:
- Loads a file with regular, named subroutine definitions.
- Subroutines become available in the main scope.
- File typically ends with ‘1;’ for successful loading indication.
- Loading a single anonymous function:
- Loads a file containing only one anonymous subroutine.
- The entire file is the sub definition, no ‘1;’ at the end.
- Returns the anonymous sub as a code reference.
- The function is assigned to a variable and called through it.
- do {} block:
- Creates an immediate code block in the current file.
- Useful for scoping and complex expressions.
- Returns the last expression’s value.
- do if:
- Conditional execution of a block.
- Useful for compact, single-condition multiple actions.
- do while:
- Creates a loop that always executes at least once.
- Condition is checked after the first execution.
- Loading external files:
- Executes an entire Perl file.
- Returns the last expression’s value from the file.
- Inline subroutines:
- Creates and immediately executes an anonymous sub.
- Useful for creating closures or complex one-time operations.
By mastering these various uses of ‘do’, you can write more expressive, modular, and flexible Perl code. Whether you’re structuring complex logic, loading dynamic content, or creating specialized control structures, ‘do’ provides a powerful and versatile tool in your Perl programming toolkit. Understanding these distinctions will help you choose the right approach for your specific coding needs and write more efficient and maintainable Perl programs.
Copyright ©️ 2024 perl.gg